티스토리 뷰
GSON이란 JSON포맷으로 되있는 문자열 데이터를 JAVA의 오브젝트로 직렬화 및 역직렬화 해주는 라이브러리이다. 예제코드와 함께 사용법을 알아보자.
라이브러리 다운
JSON
https://mvnrepository.com/artifact/org.json/json/20201115
GSON
https://mvnrepository.com/artifact/com.google.code.gson/gson/2.8.6
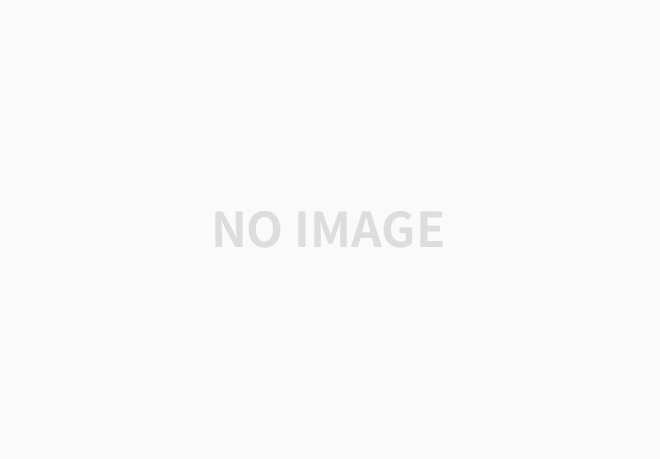
두개의 라이브러리를 다운받고 프로젝트 마우스 우클릭 > Build Path > Configure Build Path > Libraries 탭 > Add External JARs 클릭후 다운받은 jar파일을 추가한다.
JAVA Class 생성
public class Student {
private String name;
private String address;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "Student [name=" + name + ", address=" + address + "]";
}
}
JSON Object > JAVA Object
import org.json.JSONObject;
import com.google.gson.Gson;
import com.study.kjh.model.Student;
public class Main {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "kimjonghyun");
jsonObject.put("address", "Seoul");
Gson gson = new Gson();
Student student = gson.fromJson(jsonObject.toString(), Student.class);
System.out.println(student);
}
}
실행결과
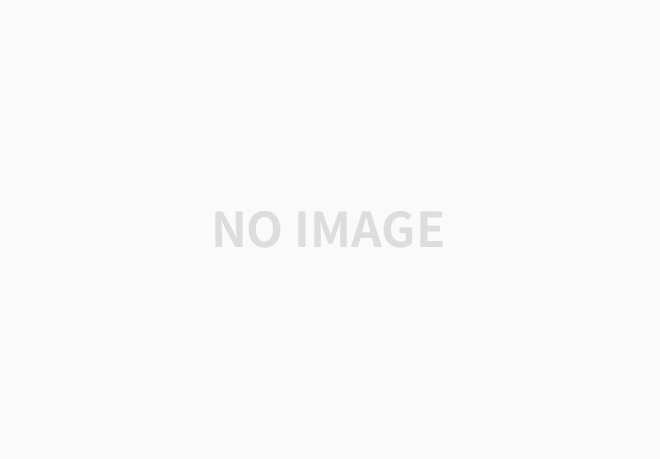
Gson객체의 fromJson() 메서드를 호출하여 첫번째인자엔 JSON포맷의 문자열, 두번째 인자엔 매핑할 티입의 Class를 적어주면 해당 Class타입의 인스턴스를 생성후 JSON 오브젝트를 인스턴스 변수의 필드와 매핑해준다.
JAVA Class 생성 (2)
public class Family {
private String name;
private String relation;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRelation() {
return relation;
}
public void setRelation(String relation) {
this.relation = relation;
}
@Override
public String toString() {
return "Family [name=" + name + ", relation=" + relation + "]";
}
}
Family Class를 생성한다.
public class Student {
private String name;
private String address;
private Family family;
public String getName() {
return name;
}
public Student() {
this.family = new Family();
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Family getFamily() {
return family;
}
public void setFamily(Family family) {
this.family = family;
}
@Override
public String toString() {
return "Student [name=" + name + ", address=" + address + ", family=" + family + "]";
}
}
Student Class의 Family타입의 필드를 추가한다.
import org.json.JSONObject;
import com.google.gson.Gson;
import com.study.kjh.model.Student;
public class Main {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "kimjonghyun");
jsonObject.put("address", "Seoul");
JSONObject family = new JSONObject();
family.put("name", "kjh");
family.put("relation", "father");
jsonObject.put("family", family);
Gson gson = new Gson();
Student student = gson.fromJson(jsonObject.toString(), Student.class);
System.out.println(student);
}
}
JSON Obejct의 value값에다 JSON Object를 추가하게되면 추가한것도 같이 JAVA Object로 변환해준다.
JSON Object의 key값과 변환할 Class의 매핑할 Field의 이름을 동일하게 적어줘야 한다
실행결과 (2)
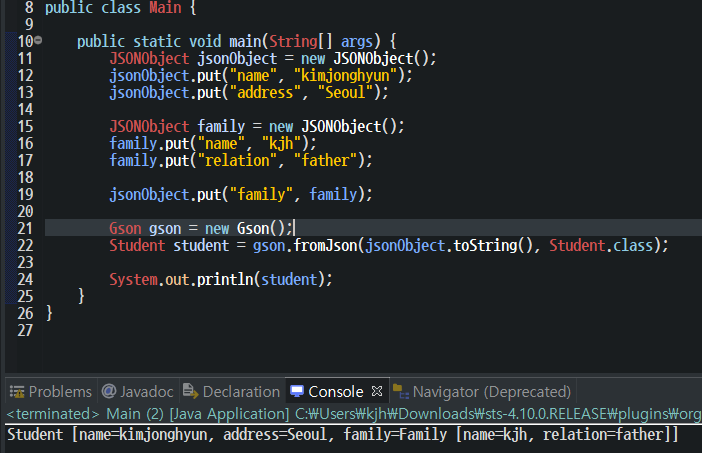
JSON Array > JAVA List
import java.lang.reflect.Type;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONObject;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import com.study.kjh.model.Student;
public class Main {
public static void main(String[] args) {
JSONArray jsonArray = new JSONArray();
String[] names = { "kimjonghyun", "kim", "jonghyun" };
String[] address = { "Seoul", "Incheon", "Busan" };
String[] relations = { "father", "mother", "sister" };
for (int i = 0; i < 3; i++) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", names[i]);
jsonObject.put("address", address[i]);
JSONObject family = new JSONObject();
family.put("name", names[i]);
family.put("relation", relations[i]);
jsonObject.put("family", family);
jsonArray.put(jsonObject);
}
jsonArray.forEach(jsonObject -> {
System.out.println("JSON Array : " + jsonObject);
});
Gson gson = new Gson();
Type type = new TypeToken<List<Student>>() {}.getType();
List<Student> students = gson.fromJson(jsonArray.toString(), type);
students.forEach(student -> {
System.out.println("JAVA List : " + student);
});
}
}
실행결과 (3)
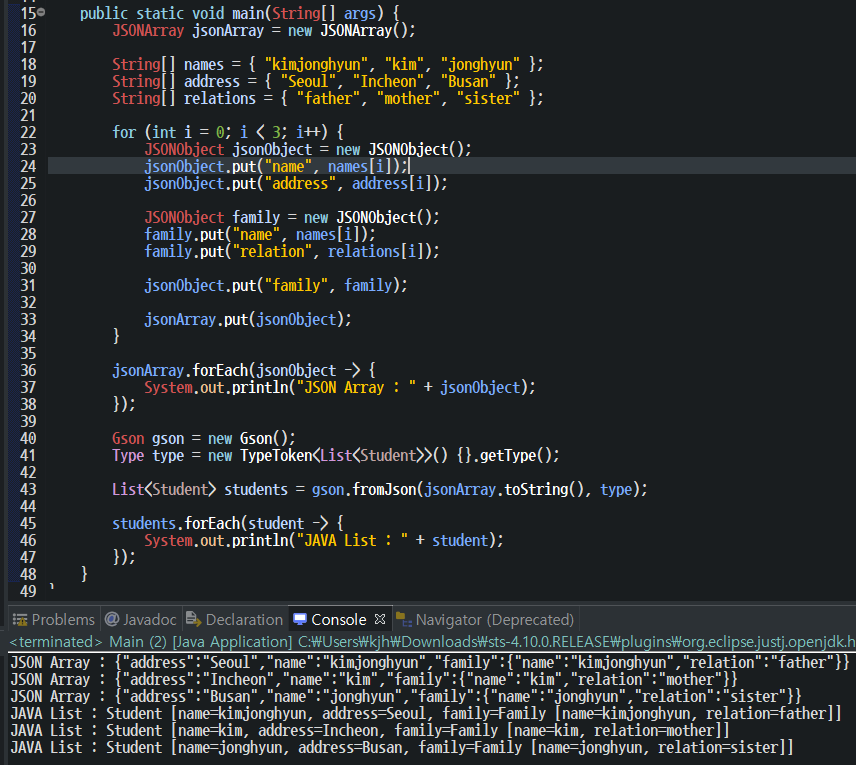
JAVA Object or List > JSON Object or Array
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.study.kjh.model.Family;
import com.study.kjh.model.Student;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
Student student = new Student();
student.setName("kimjonghyun");
student.setAddress("Seoul");
Family family = new Family();
family.setName("kjh");
family.setRelation("father");
student.setFamily(family);
// JAVA Object > JSON Object
String jsonObjectString = gson.toJson(student);
System.out.println("JSON Object : " + jsonObjectString);
List<Student> students = Arrays.asList(student);
// JAVA List > JSON Array
String jsonArrayString = gson.toJson(students);
System.out.println("JSON Array : " + jsonArrayString);
}
}
Gson객체의 toJson() 메서드를 호출하고 파라미터로 인스턴스를 넘겨주면 해당 JSON 포맷의 String 객체를 리턴한다.
실행결과 (4)
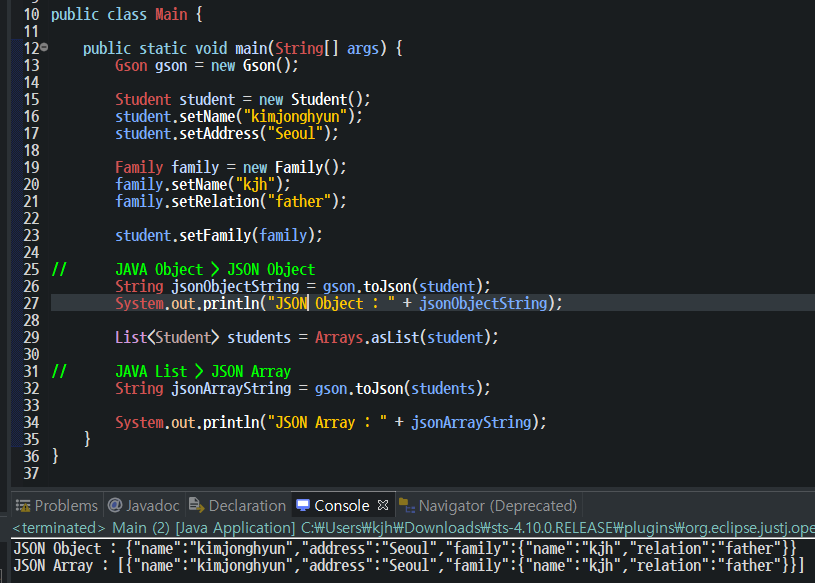
'JAVA' 카테고리의 다른 글
[JAVA] - Call By Value vs Call By Reference (3) | 2021.10.09 |
---|---|
[JAVA] - Retrofit을 활용하여 HTTP API 개발하기 (2) | 2021.09.27 |
[JAVA] - String이란? (0) | 2021.05.04 |
Reflection을 이용한 VO <-> Map 양방향 변환 (14) | 2021.04.01 |
[JAVA] - static이란? (0) | 2021.03.01 |